Project Idea due by 11:59pm Thursday.
PS4 Postmortem
Yesterday's SSL Bug
SSL Heartbeat Bug
Diagnosis of the OpenSSL Heartbleed Bug
This is the buggy version of the code (excerpted):
int
dtls1_process_heartbeat(SSL *s)
{
unsigned char *p = &s->s3->rrec.data[0], *pl;
unsigned short hbtype;
unsigned int payload;
unsigned int padding = 16; /* Use minimum padding */
/* Read type and payload length first */
hbtype = *p++;
n2s(p, payload);
pl = p;
...
if (hbtype == TLS1_HB_REQUEST)
{
unsigned char *buffer, *bp;
int r;
/* Allocate memory for the response, size is 1 byte
* message type, plus 2 bytes payload length, plus
* payload, plus padding
*/
buffer = OPENSSL_malloc(1 + 2 + payload + padding);
bp = buffer;
/* Enter response type, length and copy payload */
*bp++ = TLS1_HB_RESPONSE;
s2n(payload, bp);
memcpy(bp, pl, payload);
bp += payload;
/* Random padding */
RAND_pseudo_bytes(bp, padding);
r = dtls1_write_bytes(s, TLS1_RT_HEARTBEAT, buffer, 3 + payload + padding);
if (r >= 0 && s->msg_callback)
s->msg_callback(1, s->version, TLS1_RT_HEARTBEAT,
buffer, 3 + payload + padding,
s, s->msg_callback_arg);
OPENSSL_free(buffer);
if (r < 0)
return r;
}
...
How many serious security bugs remain in OpenSSL? (Hint: if you answer 0 will most likely be proved wrong within a month or two!)
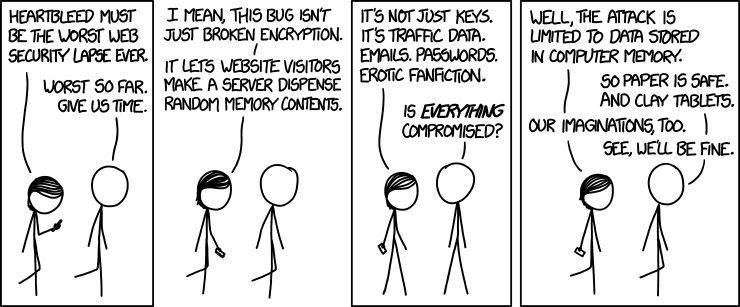
Projects
Thursday, 11:59pm (10 April): Project Idea
16-21 April: Project Reviews
24 and 29 April: Project Presentations (in class)
Do something that is fun (for you to do, and others to see), relevant (to the class), technically interesting (to you and me), and useful (at least to you, hopefully to many). You probably can’t maximize all of these! It is okay to sacrifice one or two of them to increase others. A good project should be strong on at least 2 of these, which is much better than being mediocre of all four.
You may use the projects from last semester for inspiration, but do not feel limited by the kinds of projects students did last semester, and you also have PS4 to build on.
Synchronization
E. W. Dijkstra, Solution to a Problem in Concurrent Program Control, Communications of the ACM, 1965. (1 page)
Requirements
- Only one thread may be in the critical section at any time.
- Each must eventually be able to enter its critical section.
- Must be symmetrical (all run same program).
- Cannot make any assumptions about speed of threads.
loop {
b[i] := false
L1: if k != i
c[i] := true
if b[k]:
k := i
goto L1
else:
c[i] := false
for j in [1, ..., N]:
if j != i and not c[j]:
goto L1
critical section;
c[i] := true
b[i] := true
}
How can you prove Dijkstra's solution is safe?
How can you prove Dijkstra's solution provides liveness?
How does Collab solve the mutual exclusion problem?
Leslie Lamport, A New Solution of Dijkstra's Concurrent Programming Problem, Communications of the ACM, 1972. (2 pages)
What are the advantages of Lamport's solution over Dijkstra's?