Action Items
Problem Set 2 is now posted, and is due Sunday, 9 February. Please don't wait to get started!
Read Process API and Direct Execution from OSTEP.
(Human) Communication Channels
Use IRC for immediate responses
and conversations. You should use the cs4414
channel for
course-related questions, but take advantage of the expertise on the
rust
channel also for Rust language questions. Getting direct answers
to your questions from people working on developing the language can be
even better than having polished documentation!
Use the course site for more permanent questions and discussions. Most pages (including thie one) will have their own discussion at the bottom of the page for comments relevant to that page. For general course discussions, use the Forum. For totally open discussion, use the Open Discussion Forum. It is general best if you post using your real identity on the course discussions, but if you prefer to post anonymously you should feel free to do that also.
Use email ([email protected]) for communications that don't belong in the other places including things that should be kept private (e.g., issues with your project teammate) as well as messages to notify me if you didn't get a helpful response from a question posted on the course site within a reasonable time.
Finding Rust Documentation
Rust is not mature enough to have extensive documentation yet, but (at least compared to last semester) there is now quite a bit of useful documentation.
Here are some links to documentation you may find useful (and at least one you most certainly will not):
- Rust Manual
- Rust Tutorials_NG
- Rust Cheatsheet (answers to common How to questions)
- Rust for Rubyists (mostly useful even if you don't speak Ruby)
- Rust Reddit
- Stack Overflow (Rust tag)
- Strings in Rust (tutorial from last semester)
- You can use DuckDuckGo, Bing, or Google to search the rust site by adding
site:rust-lang.org
to your queries.
(If you find other useful things, please post them in the comments below.)
Slides
Statements and Expressions
Hypothesized Rust grammar (the Rust manual has a grammar, but "looking for consistency in the manual's grammar is bad: it's entirely wrong in many places"):
IfExpression ::= if Expression Block [ else Block ]
Block ::= { [ Statement* Expr] }
Expression ::= Block
How is the meaning of ;
different in Rust and Java?
Higher-Order Functions
We can make new functions directly:
LambdaExpression ::= | Parameters | Block
Example code:
fn double(a: int) -> int { a * 2 }
fn ntimes(f: proc(int) -> int, times: int) -> proc(int) -> int {
proc(x: int) {
match times {
0 => { x }
_ => { ntimes(f, times - 1)(f(x))}
}
}
}
fn main() {
let quadruple = ntimes(double, 2);
println!("quad: {:d}", quadruple(2));
}
(This should work, but encounters a bug with Rust 0.9.)
If you are uncomfortable with code that passes procedures as parameters, I would (unbiasedly, of course) encourage you to read Chaters 4 and 5 from my [Introduction to Computing: Explorations in Language, Logic, and Machines] book. We won't spend more time explicitly covering it in this class, but you will definitely need to write code that uses higher-order procedures effectively in this class (and to be an empowered programmer in general).
Processes
What is a process?
Some terminology (not really important, but you'll hear people use them):
- Multiprogramming - program gets to run until it gets stuck, then supervisor takes over and selects another program to run.
- Non-pre-emptive multi-tasking (sometimes called co-operative multi-tasking - program gets to run until it voluntarily gives control back to the supervisor. (Sometimes used to mean the programs are all scheduled statically to recieve a particular processing time slice.)
- Normal (pre-emptive) multi-tasking - program gets to run until (approximately) supervisor decides its someone else's turn.
(For historical reasons, the terms and hyphenating-conventions are confusing, using "program", "task", and "process" to essentially interchangably, except that the historical term multiprogramming is different from multitasking.)
What are some consequences of the difference between non pre-emptive multi-tasking and pre-emptive multi-tasking?
How can pre-emptive multi-tasking even be possible with only one processor?
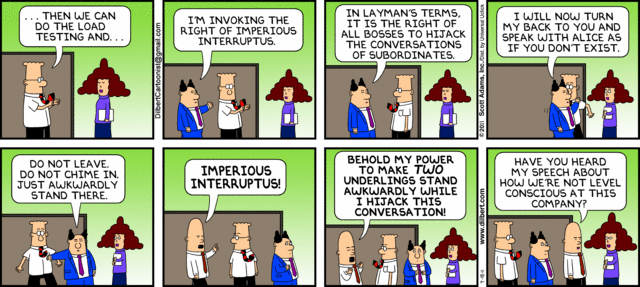
How often should the kernel timer interrupt (a.k.a., "supervisor's alarm clock") go off?
What programs should be able to change the kernel timer interrupt frequency?
Who prefers the kernel timer interrupt interval to be shorter? Who prefers it to be longer?
Wil Thomason's Kernel Timer Interrupt program (for measuring the kernel timer interrupt time on your machine). (This worked in Rust 0.8, you may need to make some changes to update it to work in Rust 0.9.)
Links and Videos
Here's the movie clip I didn't show in class about how Mac OS X got preemptive multi-tasking:
If you missed Sorkin's earlier moving about operating systems, here's the key scene from that one:
For a more truthful account of how Mark did in his Operating Systems course, see Matt Welsh's How I almost killed Facebook. This is the lecture from the movie - we'll get into virtual memory some later in the course, but the lecture in the movie is pretty good if you want to get a head start. ("The take-away from the movie is clear: nerds win. Ideas are cheap and don't mean squat if you don't know how to execute on them. To have an impact you need both the vision and the technical chops, as well as the tenacity to make something real." - Matt Welsh on The Social Network.)